1.重点!题目给出用栈进行中序遍历的操作,要求还原二叉树,并层序遍历。
2.如果上次没有弹出,并且栈为空,则这次压入的为根。
3.如果上次有弹出,并且这次压入,那么上次弹出的是父节点,这次压入的是右子节点。
4.如果上次没有弹出,并且这次压入,那么这次压入的是栈头的左子节点。
5.每次弹出一个节点,都要把这个节点记录下来,lastPop。
An inorder binary tree traversal can be implemented in a non-recursive way with a stack. For example, suppose that when a 6-node binary tree (with the keys numbered from 1 to 6) is traversed, the stack operations are: push(1); push(2); push(3); pop(); pop(); push(4); pop(); pop(); push(5); push(6); pop(); pop(). Then a unique binary tree (shown in Figure 1) can be generated from this sequence of operations. Your task is to give the postorder traversal sequence of this tree.
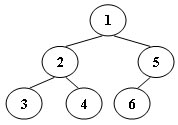
Each input file contains one test case. For each case, the first line contains a positive integer N (<=30) which is the total number of nodes in a tree (and hence the nodes are numbered from 1 to N). Then 2N lines follow, each describes a stack operation in the format: “Push X” where X is the index of the node being pushed onto the stack; or “Pop” meaning to pop one node from the stack.
Output Specification:
For each test case, print the postorder traversal sequence of the corresponding tree in one line. A solution is guaranteed to exist. All the numbers must be separated by exactly one space, and there must be no extra space at the end of the line.
Sample Input:
6 Push 1 Push 2 Push 3 Pop Pop Push 4 Pop Pop Push 5 Push 6 Pop Pop
Sample Output:
3 4 2 6 5 1
AC代码:
//#include<string> //#include <iomanip> //#include<stack> //#include<unordered_set> //#include <sstream> //#include "func.h" //#include <list> #include<unordered_map> #include<set> #include<queue> #include<map> #include<vector> #include <algorithm> #include<stdio.h> #include<iostream> #include<string> #include<memory.h> #include<limits.h> #include<stack> using namespace std; struct TreeNode { int val; TreeNode*l, *r; TreeNode() :val(-1), l(NULL), r(NULL){}; TreeNode(int x) :val(x), l(NULL), r(NULL){}; }; void InOrder(TreeNode*root, vector<int>&in) { if (root) { InOrder(root->l, in); InOrder(root->r, in); in.push_back(root->val); } } int main(void) { stack<TreeNode*> sta; TreeNode*root = NULL; TreeNode*lastPop = NULL; int n; cin >> n; for (int i = 0; i < 2 * n; i++) { string str; cin >> str; if (str == "Push") { int tmp; cin >> tmp; if (sta.empty() && lastPop == NULL) { root = new TreeNode(tmp); sta.push(root); } else if (lastPop) {//如果上次pop出了,这次压入,证明是右子树 lastPop->r = new TreeNode(tmp); sta.push(lastPop->r); } else { sta.top()->l = new TreeNode(tmp); sta.push(sta.top()->l); } lastPop = NULL;//这次是压入,所以没有上次Pop出的元素的值 } else {//这次是pop出,所以需要存储pop出的元素 TreeNode*head = sta.top(); sta.pop(); lastPop = head; } } vector<int> in(0); InOrder(root, in); for (int i = 0; i < in.size(); i++) { cout << in[i]; if (i != in.size() - 1) cout << " "; } cout << endl; return 0; }