1.题目给出一棵和一个权重值W,要求求出从根到叶子节点权重累加为W的路径。
2.该题使用树节点数据结构,其中包含vector<int> child列表和权重值。
3.使用DFS进行遍历搜索(节点最多100个),能够满足要求。
Given a non-empty tree with root R, and with weight Wi assigned to each tree node Ti. The weight of a path from R to L is defined to be the sum of the weights of all the nodes along the path from R to any leaf node L.
Now given any weighted tree, you are supposed to find all the paths with their weights equal to a given number. For example, let’s consider the tree showed in Figure 1: for each node, the upper number is the node ID which is a two-digit number, and the lower number is the weight of that node. Suppose that the given number is 24, then there exists 4 different paths which have the same given weight: {10 5 2 7}, {10 4 10}, {10 3 3 6 2} and {10 3 3 6 2}, which correspond to the red edges in Figure 1.
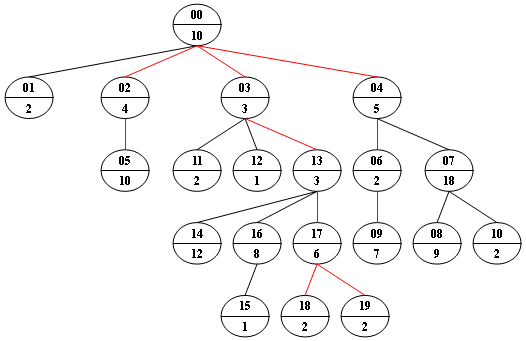
Figure 1
Each input file contains one test case. Each case starts with a line containing 0 < N <= 100, the number of nodes in a tree, M (< N), the number of non-leaf nodes, and 0 < S < 230, the given weight number. The next line contains N positive numbers where Wi (<1000) corresponds to the tree node Ti. Then M lines follow, each in the format:
ID K ID[1] ID[2] ... ID[K]
where ID is a two-digit number representing a given non-leaf node, K is the number of its children, followed by a sequence of two-digit ID’s of its children. For the sake of simplicity, let us fix the root ID to be 00.
Output Specification:
For each test case, print all the paths with weight S in non-increasing order. Each path occupies a line with printed weights from the root to the leaf in order. All the numbers must be separated by a space with no extra space at the end of the line.
Note: sequence {A1, A2, …, An} is said to be greater than sequence {B1, B2, …, Bm} if there exists 1 <= k < min{n, m} such that Ai = Bifor i=1, … k, and Ak+1 > Bk+1.
Sample Input:
20 9 24 10 2 4 3 5 10 2 18 9 7 2 2 1 3 12 1 8 6 2 2 00 4 01 02 03 04 02 1 05 04 2 06 07 03 3 11 12 13 06 1 09 07 2 08 10 16 1 15 13 3 14 16 17 17 2 18 19
Sample Output:
10 5 2 7 10 4 10 10 3 3 6 2 10 3 3 6 2
AC代码:
//#include<string> //#include<stack> //#include<unordered_set> //#include <sstream> //#include "func.h" //#include <list> #include <iomanip> #include<unordered_map> #include<set> #include<queue> #include<map> #include<vector> #include <algorithm> #include<stdio.h> #include<iostream> #include<string> #include<memory.h> #include<limits.h> #include<stack> using namespace std; struct TreeNode{ vector<int> child; int w; TreeNode() :child(0), w(0){}; }; void dfs(int now, int target, vector<TreeNode>&tree, vector<int>&path, vector<vector<int>>&ans) { if (tree[now].child.size() == 0 && target == 0) ans.push_back(path);//叶子节点,并且满足要求 if (tree[now].child.size() != 0 && target <= 0) return;// else if (target < 0) return; for (int i = 0; i < tree[now].child.size(); i++) { path.push_back(tree[tree[now].child[i]].w); dfs(tree[now].child[i], target - tree[tree[now].child[i]].w, tree, path, ans); path.pop_back(); } } bool cmp(const vector<int>&a, const vector<int>&b) { for (int i = 0; i < min(a.size(), b.size()); i++) { if (a[i] >b[i]) return true; else if (a[i] < b[i]) return false; } return false; } int main(void) { int nodeSum, nonLeafSum, target; cin >> nodeSum >> nonLeafSum >> target; vector<TreeNode> tree(nodeSum); for (int i = 0; i < nodeSum; i++) { scanf("%d", &tree[i].w); } for (int i = 0; i < nonLeafSum; i++) { int nodeNum=0; scanf("%d", &nodeNum); int childSum = 0; scanf("%d", &childSum); tree[nodeNum].child = vector<int>(childSum); for (int j = 0; j < childSum; j++) { scanf("%d", &tree[nodeNum].child[j]); } } if (nodeSum > 0) { vector<int> path; vector<vector<int>> ans; path.push_back(tree[0].w);//注意判断根节点是否存在 target -= tree[0].w; dfs(0, target, tree, path, ans); sort(ans.begin(), ans.end(), cmp); for (int i = 0; i < ans.size(); i++) { for (int j = 0; j < ans[i].size(); j++) { printf("%d", ans[i][j]); if (j != ans[i].size() - 1) printf(" "); } printf("\n"); } } return 0; }